What is PHP?
PHP (Hypertext Preprocessor) is a server-side language designed for web development. Within an HTML page, you can write PHP codes that will be processed at the server. HTML is generated after the PHP codes are processed and sent to the web browser as the output.
PHP Tags
PHP codes begin with <?php and ends with ?>. If you have created HTML pages, you would find this syntax familiar, being that HTML tags begin with a less than symbol (<) and ends with a greater than symbol (>). These opening and closing PHP tags tell the webserver where the PHP code execution should start and finish. Any text outside these tags is treated as normal HTML.
PHP statements
PHP statements are written inside the opening and closing tags.
eg:
<?php
echo "PHP tutorial";
?>
The code above outputs PHP tutorial on your web browser.
Comments
Comments are used to document codes. Comments can be used to explain your codes to other programmers. Any text that is commented is ignored by the PHP interpreter. There are two ways to comment in PHP:
1. Multiline comments
<?php
/*
Here I used a for loop to
get the sum of all the integers
*/
?>
2. Single line comments
<?php
//this is a comment
?>
Variables
A variable is a storage area where you can store a value that can be used at a later time. Variables act as a container that holds values that can be referenced later. Think of variables as buckets that can hold items that can be retrieved when needed.
Identifiers
Identifiers are the names of variables. To name a variable, there are some simple rules you should follow:
- Variable names/identifiers can have any length and can consist of letters, numbers and underscores.
- Variable names/identifiers cannot start with a digit.
-Variable names/identifiers are case sensitive. $firstname is not the same as $FirstName.
Creating a variable
To create a variable, use the dollar sign and the name or the identifier of the variable.
eg: $firstname
$age
$result
Assigning values to variables
To assign values to variables use the assignment operator (=)
eg:
$firstname="John";
$age=21;
$result=4.92;
Variable types
A variable’s type refers to the kind of data that is being stored in it.
PHP data types
PHP supports the following data types:
- Integer: used for whole numbers.
- Double: used for real numbers.
- String: used for strings of characters.
- Boolean: used for true or false values.
- Array: used to store multiple data items of the same type.
- Object: used for storing instances of classes
- Null: variables that have not been given a value or have been unset
- Resource: certain built-in function (such as database functions) returns variables that have the type resource. These types of variables are usually used internally which means that you won't/shouldn't alter them.
Constants
A constant stores a value such as a variable. The difference between a variable and constant is that in a constant, once its value is set, it cannot be changed.
To define a constant, use this function:
define(‘SUM’, 300);
The names of constants are written in uppercase. This is not a must. It is just a convention to make it easy to differentiate between variables and constants.
Variable scope
Scope refers to the places within a PHP script where a particular variable is visible and can be accessed. The three types of scope in PHP are as follows:
- Global/superglobal
- Local variables
- Static variables
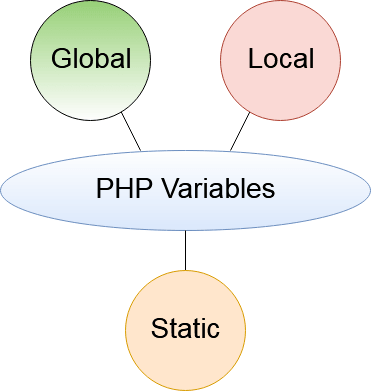
The arrays $_GET and $_POST and some other special variables have their own scope rules. These are known as superglobals and can be accessed and seen everywhere, both inside and outside methods or functions.
The complete list of superglobals is as follows:
- $GLOBALS: an array of all global variables.
- $_SERVER: an array of server environment variables.
- $_GET: an array of variables passed to the script via the GET method.
- $_POST: an array of variables passed to the script via the POST method.
- $_COOKIE: an array of cookie variables.
- $_FILES: an array of variables related to file uploads.
- $_ENV: an array of environment variables.
- $_REQUEST: an array of all user input variables.
- $_SESSION: an array of all session variables.